The following example script describes how to monitor Cisco IPsec Phase-1 IKE Site-to-Site Tunnel status. The monitor observes the tunnels provided while assigning a template. Apply scripts on each device individually and not through device management policy.
Script - example
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import groovy.transform.CompileStatic;
import com.vistara.gateway.plugin.snmp.monitor.SnmpExtendedAPI;
class CiscoIpSecTunnelStatus {
private static final String VPN_TUNNEL_STATUS = (String) "cisco.vpn.ike.tunnel.status";
private static final String VPN_REMOTE_PEER_IDENTITY = (String) "cisco.vpn.remote.peer.identity";
private static final String VPN_REMOTE_PEER_NAME = (String) "cisco.vpn.name";
private static final String VPN_DOWN_STATUS = (String) "2";
private static final String VPN_UP_STATUS = (String) "1";
private static final String EMPTY_STRING = (String) "";
private static final String VPN_TUNNEL_REMOTE_PEER_TABLE = (String) "1.3.6.1.4.1.9.9.171.1.2.3.1.7";
@CompileStatic
void execute(SnmpExtendedAPI api) throws Exception {
HashSet<String> currentPeers = new HashSet<>();
HashMap<String, String> reqVpnEntries = new HashMap<>();
/*
* Step 1: Fetching user given component inputs using API Call and
* prepares reqVpnEntries hash with VPN_REMOTE_PEER_IP as key and
* VPN_REMOTE_PEER_NAME as value
*
*/
List<HashMap<String, String>> compList = (List<HashMap<String, String>>) api.getComponentScopeMap();
if (compList == null || compList.size() <= 0)
return;
for (int i = 0; i <= compList.size() - 1; i++) {
HashMap<String, String> compMap = (HashMap<String, String>) compList.get(i);
if (compMap != null && compMap.size() > 0) {
String compRemoteIp = (String) compMap.get(VPN_REMOTE_PEER_IDENTITY);
String compName = (String) compMap.get(VPN_REMOTE_PEER_NAME);
if (compName == null || EMPTY_STRING.equals(compName) || compRemoteIp == null || EMPTY_STRING.equals(compRemoteIp)){
continue;
}
reqVpnEntries.put(compRemoteIp, compName);
}
}
/*
* Step 2: SNMPWALK for IKE Remote Peer IP table and prepares
* currentPeers hashset
*
*/
HashMap<String, String> resultant = (HashMap<String, String>) api.getSnmpTable(VPN_TUNNEL_REMOTE_PEER_TABLE);
if (resultant != null){
for (String peerVariable : resultant.values()) {
if (peerVariable != null && !peerVariable.isEmpty()) {
currentPeers.add(peerVariable);
}
}
}
for (String reqVpnIP : reqVpnEntries.keySet()) {
if (currentPeers.contains(reqVpnIP)) {
HashMap<String, String> temp = new HashMap<>();
temp.put(VPN_TUNNEL_STATUS, VPN_UP_STATUS); // Here: 1=>OK and
// 2=>Critical
temp.put(VPN_REMOTE_PEER_IDENTITY, reqVpnIP);
api.addOutputMetric(reqVpnEntries.get(reqVpnIP), temp);
} else {
HashMap<String, String> temp = new HashMap<>();
temp.put(VPN_TUNNEL_STATUS, VPN_DOWN_STATUS); // Here: 1=>OK andG
// 2=>Critical
temp.put(VPN_REMOTE_PEER_IDENTITY, reqVpnIP);
api.addOutputMetric(reqVpnEntries.get(reqVpnIP), temp);
}
}
}
}
Script description
Import libraries
Import all libraries in this section based on your requirement.
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import groovy.transform.CompileStatic;
import com.vistara.gateway.plugin.snmp.monitor.SnmpExtendedAPI;
Define a user-defined class
The user-defined class enables you to declare and initialize global variables.
Use the same metric name on the Setup > Monitoring > Monitors > Create a Monitor screen and in the script.
class CiscoIpSecTunnelStatus {
private static final String VPN_TUNNEL_STATUS = (String) "cisco.vpn.ike.tunnel.status";
private static final String VPN_REMOTE_PEER_IDENTITY = (String) "cisco.vpn.remote.peer.identity";
private static final String VPN_REMOTE_PEER_NAME = (String) "cisco.vpn.name";
private static final String VPN_DOWN_STATUS = (String) "2";
private static final String VPN_UP_STATUS = (String) "1";
private static final String EMPTY_STRING = (String) "";
private static final String VPN_TUNNEL_REMOTE_PEER_TABLE = (String) "1.3.6.1.4.1.9.9.171.1.2.3.1.7";
Implement business logic
Implement business logic using the following required function:
void execute(SnmpExtendedAPI api) throws Exception {
Do not change the method signature.
Parse user-defined input
Parse user-defined input using the following API methods depending on the component or monitor level scope:
- api.getComponentScopeMap();
- api.getComponentScopeMap(index);
- api.getMonitorScopeValue(key);
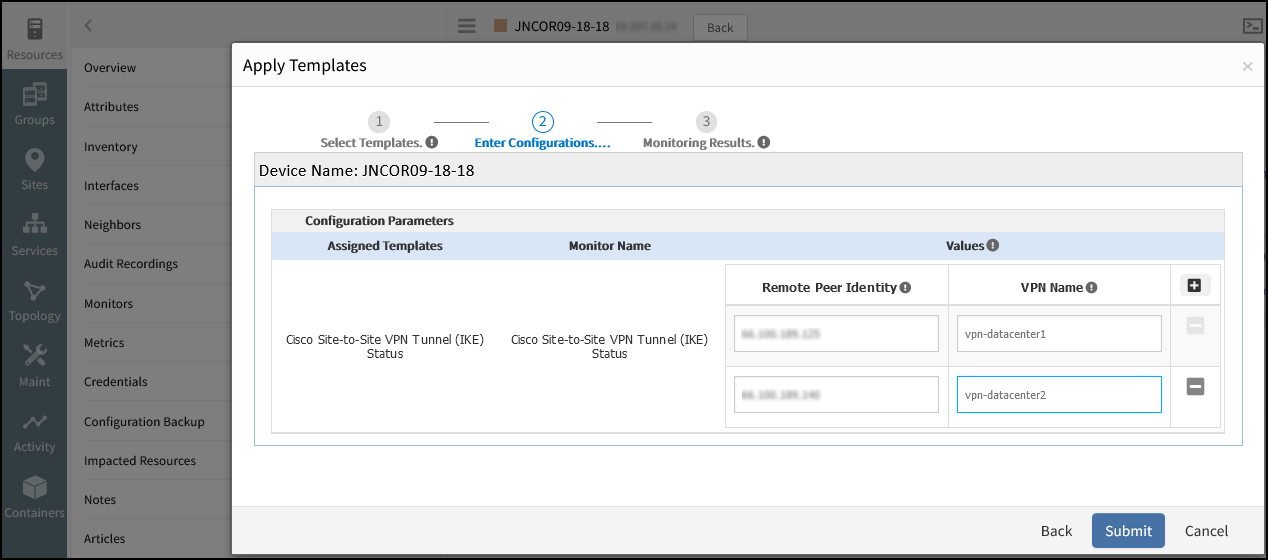
List<HashMap<String, String>> compList = (List<HashMap<String, String>>) api.getComponentScopeMap();
if (compList == null || compList.size() <= 0)
return;
for (int i = 0; i <= compList.size() - 1; i++)
{
HashMap<String, String> compMap = (HashMap<String, String>) compList.get(i);
if (compMap != null && compMap.size() > 0)
{
String compRemoteIp = (String) compMap.get(VPN_REMOTE_PEER_IDENTITY);
String compName = (String) compMap.get(VPN_REMOTE_PEER_NAME);
if (compName == null || EMPTY_STRING.equals(compName) || compRemoteIp == null || EMPTY_STRING.equals(compRemoteIp))
{
continue;
}
reqVpnEntries.put(compRemoteIp, compName);
}
}
Query SNMP OIDs
Query SNMP OIDs using one of the following API methods based on your requirement:
- api.getSnmpRequest(String sOid);
- api.getSnmpRequest(String[] sOids);
- api.getSnmpRequest(String sOid, String format);
- api.getSnmpTable(String sOid);
- api.getSnmpTable(String sOid, String format);
HashMap<String, String> resultant = (HashMap<String, String>) api.getSnmpTable(VPN_TUNNEL_REMOTE_PEER_TABLE);
Process SNMP OID results
Use one of the following API methods to store or delete previous poll values in the cache:
- api.getPersistantValue(uuid);
- api.deletePersistantValue(String uuid);
Use the following API methods to get device details into the script:
- api.getResourceIp();
- api.getResourceName();
- api.getResourceUuid();
Use the following API methods based on user requirement:
- api.getBigDecimalMetricValue(String instance, String metric);
- api.getInstanceNames();
- api.getMetrics(String instance);
- api.getStringMetricValue(String instance, String metric);
if (resultant != null)
{
for (String peerVariable : resultant.values())
{
if (peerVariable != null && !peerVariable.isEmpty())
{
currentPeers.add(peerVariable);
}
}
}
Add output metric values
Add output metric values in a standard JSON format using the following API methods based on your requirement:
- api.addOutputMetric(HashMap<String, HashMap<String, String» metricResultMap);
- api.addOutputMetric(String compName, HashMap<String, String> metricResultMap);
- api.addOutputMetric(String metric, String value);
- api.addOutputMetric(String metric, String instance, String value);
- api.addOutputMetric(String metric, String instance, int value);
- api.addOutputMetric(String metric, String instance, long value);
- api.addOutputMetric(String metric, String instance, double value);
- api.addOutputMetric(String metric, String instance, float value);
for (String reqVpnIP : reqVpnEntries.keySet())
{
if (currentPeers.contains(reqVpnIP))
{
HashMap<String, String> temp = new HashMap<>();
temp.put(VPN_TUNNEL_STATUS, VPN_UP_STATUS); // Here: 1=>OK and
// 2=>Critical
temp.put(VPN_REMOTE_PEER_IDENTITY, reqVpnIP);
api.addOutputMetric(reqVpnEntries.get(reqVpnIP), temp);
}
else
{
HashMap<String, String> temp = new HashMap<>();
temp.put(VPN_TUNNEL_STATUS, VPN_DOWN_STATUS); // Here: 1=>OK andG
// 2=>Critical
temp.put(VPN_REMOTE_PEER_IDENTITY, reqVpnIP);
api.addOutputMetric(reqVpnEntries.get(reqVpnIP), temp);
}
}